Parsing Strings into Different Data Types
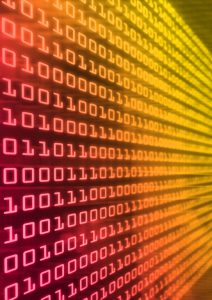
There are two fundamental things to understand about parsing strings.
- Unless another program is making the string that you’re parsing, things can always go awry (and a program may not always make it correctly either!)
- There are two different ways a string can be formatted.
- Delimited
- Fixed Width
In most cases today, a string that you’ll be parsing is delimited, with the most popular format being CSV, or Comma Separated Values. To get this standard right, all strings that are in the files need to have double quotes around them. For this reason, many modern files will use tabs for separators instead of commas, and you could even see spaces as separators.
Regardless of what is used to separate, if you’re writing code to parse this instead of just using an off the shelf tool, the Split function is your friend.
Parsing the String
Split takes a string and creates an array of strings that are separated by the character or string that you specify. For example, if I wanted to parse a string of numbers, I’d use split to get them all:
string SpaceSeparatedValues = "1 2 3 4 5 6"; // A list of values
string[] IndividualValues = SpaceSeparatedValues.Split(' '); // Split on the single space character
What you’ll end up with is an array of strings that have the values in them. The value of IndividualValues[0] will be 1, IndividualValues[1] will be 2 and so on.
But this isn’t what we really want, because these are numbers and not strings.
Changing to Numbers
We really want to change these strings to numbers, so what we have to do here is call the Parse routine on datatype we want to use:
int[] IntValues = new int[6];
for(i = 0; i < 6; i++) {
IntValues[i] = int.Parse(IndividualValues[i]);
}
This sequence will change the strings into Integers– or floats or decimals or whatever it is that you want your data to be in.
This is the basic building block of string manipulation, and you’re definitely going to deal with a lot of strings in your time as a coder whether it’s dealing with JSON, XML, and such. Fortunately, there are a lot of tools to do this type of parsing for you, but it doesn’t hurt to understand how it’s working does it?
I mean, I used to have to walk through the string array, record the location of the space and then copy off to other values. You don’t want to do that, do you?!